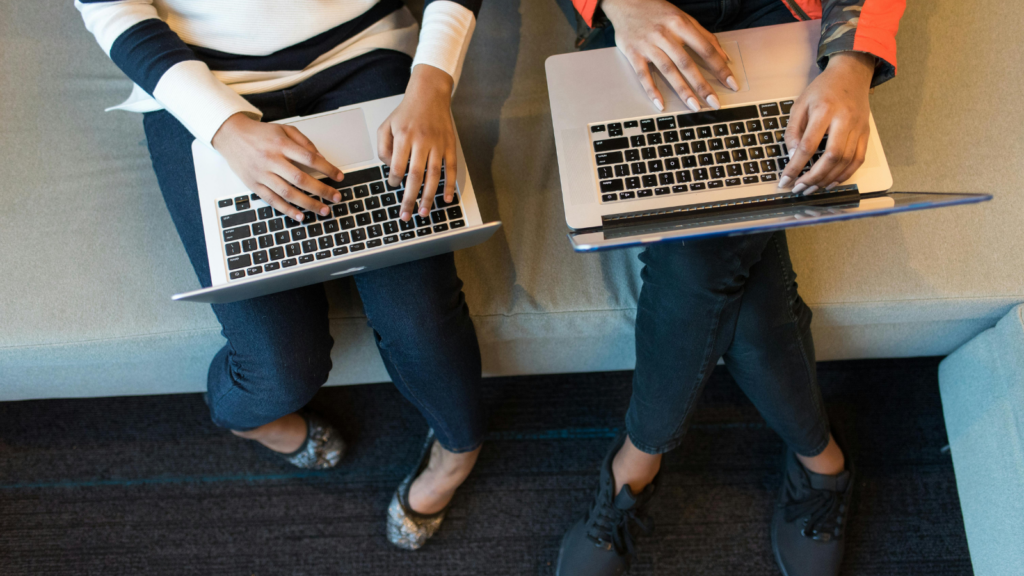
In the vast and ever-evolving world of Python programming, understanding the nuances can be the key to efficient coding. One such nuance is knowing how to check if a list is empty in Python. It’s a vital skill, whether you’re a beginner just getting your feet wet, or a seasoned programmer looking to brush up on your Python know-how.
This article will guide you through the process of how to check if a list is empty python, breaking it down into simple, easy-to-understand steps. You’ll learn the different methods available and when to use each one. So, let’s dive into the world of Python lists, and unlock the potential of efficient and effective coding.
How to Check if a List Is Empty Python
Testing for an empty list in Python entails the usage of two particular methods. The first involves using the Boolean function. Programming in Python recognizes an empty list as false, so when a list is tested in an if statement, it evaluates as “True” when there’s content, and “False” if it’s empty. Here’s an example:
my_list = []
if not my_list:
print(“List is empty”)
The second method employs the len() function. By determining the length of the list, it establishes whether or not it’s empty. A zero list length signifies that the list contains no elements. Check this example below:
my_list = []
if len(my_list)==0:
print(“List is empty”)
These vital methods establish a foundation in managing lists as an experienced programmer or a beginner in Python.
Best Practices When Checking List Emptiness
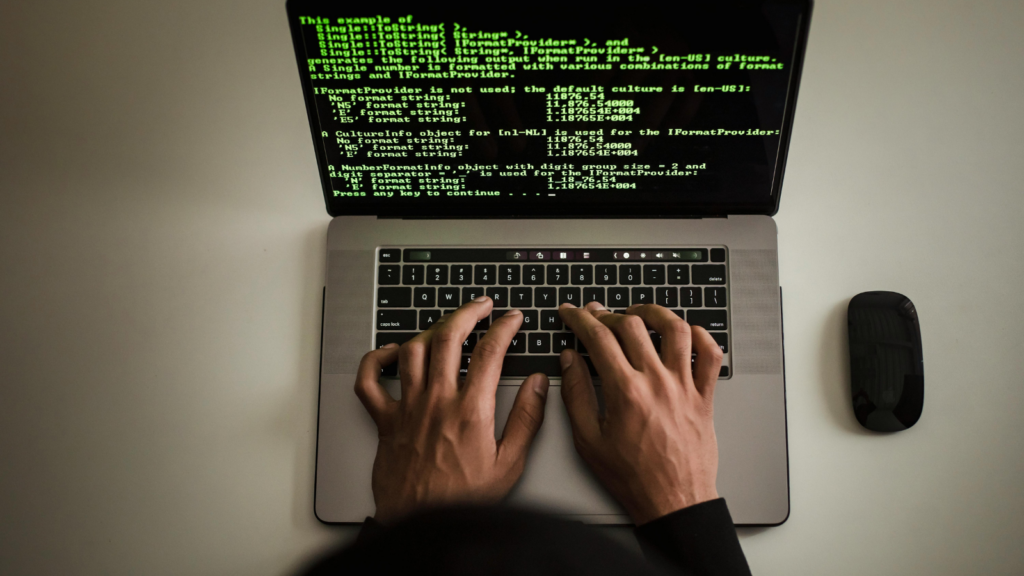
‘When examining Python lists, users often ask, how to check if a list is empty Python? While many programmers opt for either Boolean evaluation or the len() function, they should keep certain practices in mind. For instance, when using the Boolean function, they must remember that an empty Python list equates to “False”. Conversely, with the len() function, it’s vital not to confuse a Python list’s length with its content. A list’s length can be zero, indicating emptiness, even if non-null content is inside the list. These practices help programmers be more precise and avoid common pitfalls when working with Python lists. Moreover, avoiding arbitrary checks further enhances the clarity and reliability of their Python code. Utilizing these simple practices can greatly increase code efficiency and readability.
Common Mistakes to Avoid
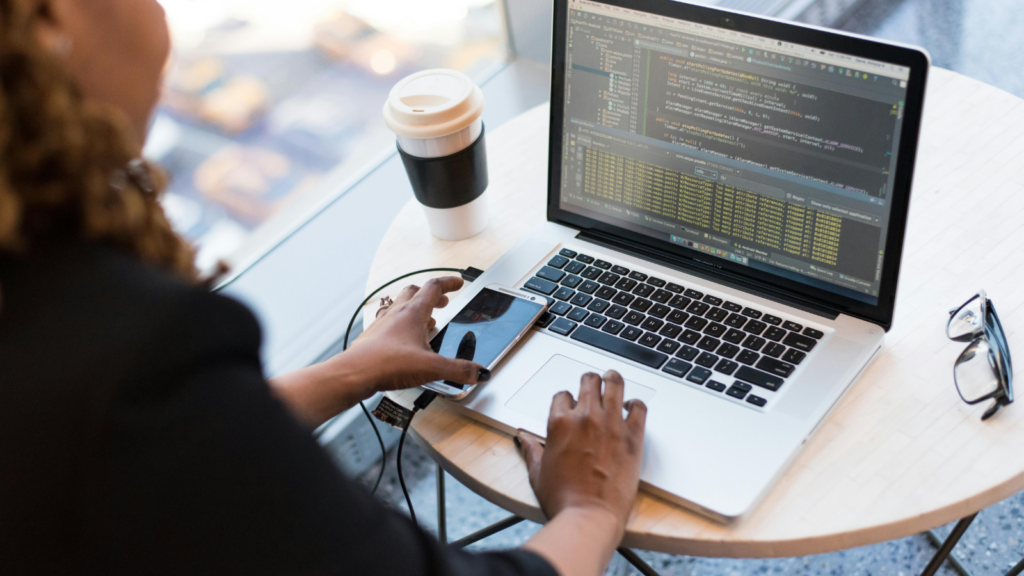
Entering a Python code journey, it’s vital to sidestep prevalent blunders when checking for empty lists. Amateurs often conflate the length of a list with its contents, an error that may compromise their coding efficiency. For example, an empty list like [] has a length of 0, but a list like [None] isn’t empty, despite its none-content. Developers must remember that length alone can’t testify to a list’s emptiness.
Likewise, another common misstep is to ignore Python’s built-in capabilities. Utilizing Boolean evaluation or the len() function provides a direct, convenient means of determining if a list is empty. Brushing off such useful tools and crafting custom methods may result in redundant and inefficient code.
Finally, assuming all non-empty lists are useful can lead down a wrong path. It’s possible for a list in Python to contain- null, whitespace, or otherwise non-useful data. Therefore, efficient code check doesn’t just ensure the list isn’t empty, but also that the contents are meaningful to the task at hand.
Avoiding these common mistakes when determining how to check if a list is empty python, programmers can significantly bolster their code’s reliability, clarity, and efficiency.
A Game Changer for All Programmers
Mastering the art of how to check if a list is empty python is a game-changer for any programmer. It’s not just about knowing the methods like Boolean evaluation and the len() function, but also about avoiding common pitfalls. They’ve learned that a list’s length doesn’t necessarily reflect its contents and that Python’s built-in capabilities should never be overlooked. Creating custom methods may seem tempting, but it often leads to unnecessary and inefficient code. They now understand the importance of ensuring non-empty lists carry meaningful data. With these insights, they’re equipped to write Python code that’s more reliable, clear, and efficient. They’ve taken another step towards becoming a more proficient Python programmer.