[ez-oc]
Diving into the world of Python programming, one often stumbles upon the concept of Python string replace regex. It’s a powerful tool, a game-changer for handling and manipulating text data. Yet, its full potential remains untapped by many, mainly due to its perceived complexity.
This article will demystify Python string replace regex, breaking it down to its core components. Whether you’re a seasoned coder looking to refine your skills, or a newbie seeking to grasp the basics, you’re in the right place.
Python String Replace Regex
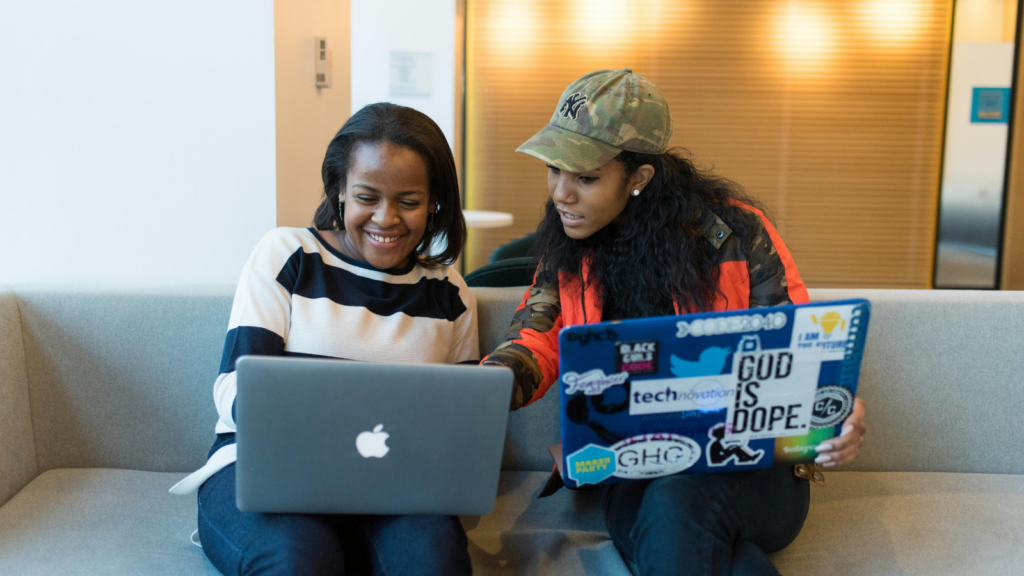
Delving deeper into string replacement with regex in Python, it’s crucial to remember that Python’s re module provides regular expression matching operations incredibly similar to those found in Perl. Here, two particular methods, re.sub() and re.subn(), are employed for replacing strings.
re.sub(pattern, repl, string, count=0, flags=0), where pattern is the regular expression to be matched, repl is the string to replace occurrences with, string is the original string, and count and flags are optional parameters. If count is greater than zero, it controls the maximum number of pattern occurrences to be replaced. Otherwise, all occurrences will be replaced.
re.subn() possesses a similar syntax but offers a different return type. It brings back a tuple with the new string and the number of substitutions made.
Let’s cite a basic example that illustrates the practical use of these methods:
import re
text = “Python is fun.”
new_text = re.sub(r”\bPython\b”, “Coding”, text)
print(new_text)
In this example, “Python” is replaced with “Coding” using re.sub(), resulting in “Coding is fun.”
Practical Examples of Python String Replace Regex
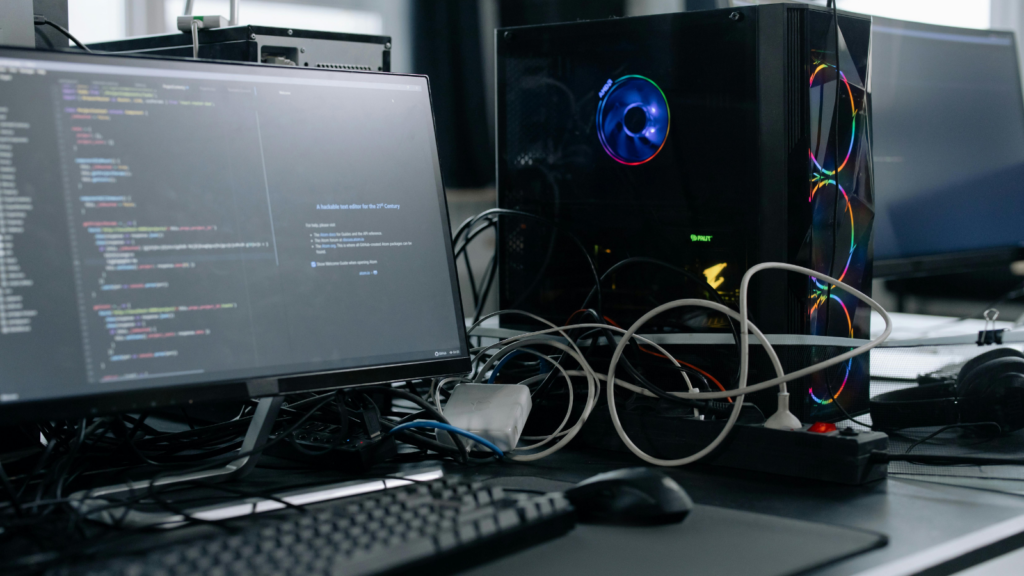
Building on the knowledge of Python string replace regex previously discussed, let’s delve into some practical examples. They’ll provide a clear understanding of Python’s powerful re module and its efficiency in handling complex text-based data.
- Removing Digits from a String
This example involves using regex to remove all numeric digits from a text. The method for this operation is re.sub(“\d”, “”, text), which instructs Python to replace any digit (denoted by “\d”) in the string with nothing. - Reformatting a Date String
Python string replace regex even works for reformatting date strings, demonstrating its functionality with more intricate patterns. Suppose one has a date in the format ‘dd-mm-yyyy’ and wants to change it to ‘yyyy/mm/dd’. The execution would be re.sub(“(\d{2})-(\d{2})-(\d{4})”, r”\3/\2/\1″, date). - Replacing Multiple Substrings
In a situation where there’s a need to substitute multiple different substrings within a text, Python’s re.sub() excels. For instance, if “cat” and “dog” are to be swapped in a string, a dictionary of replacements ({‘cat’: ‘dog’, ‘dog’: ‘cat’}) and a function within re.sub() combine to perform the task.
Performance Tips for Regex in Python
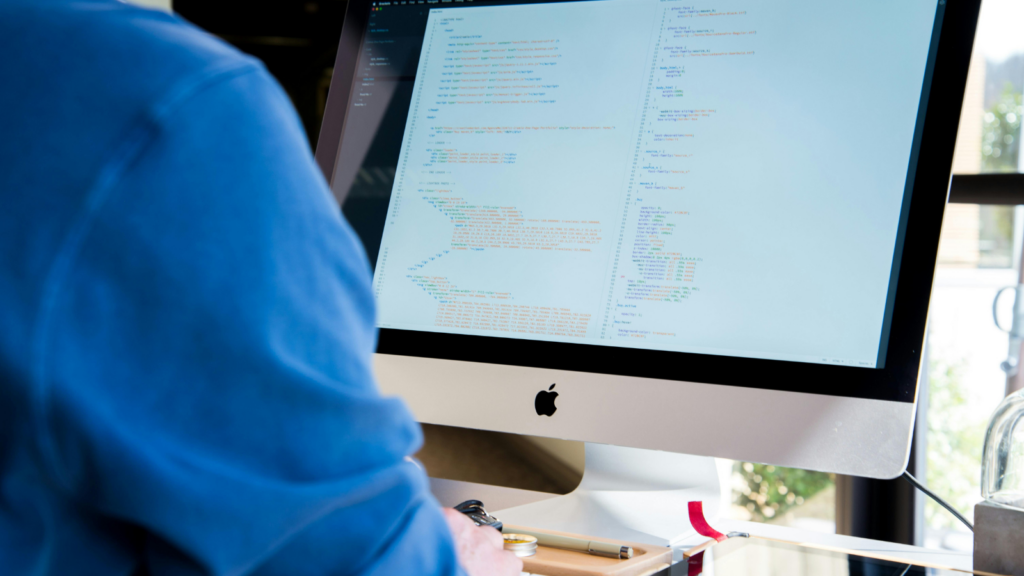
Primarily, applying python string replace regex in a competent manner benefits efficiency. An essential tip remains compile regular expressions where frequent usage is a requirement. This action, by using re.compile(), stores the regex pattern object, available for later use, drastically reducing the overall program execution time.
Secondly, strategic use of special characters, such as ‘\b’, enhances the precision regarding match targets. Its inclusion restricts the regex patterns to a word boundary, averting unnecessary, broad matches.
Next, employing non-capturing groups, for instance ‘(?:regex)’, also significantly enhances speed. It prevents Python from storing backreferences, ideal for patterns repeated in the string, and optimizes regex performance by reducing memory overheads.
Additionally, harness ‘re.IGNORECASE’ or its equivalent flag, ‘re.I’, in relevant scenarios. Forcing case insensitivity, it narrows down the pattern diversity, optimizing the matching process.
Lastly, apply raw strings, ‘r’ prefix to regex patterns, to avoid backslashes creating escape sequences, ensuring a more reliable regex pattern execution.
Powerful Tool for Text Manipulation
Python’s string replace regex is a powerful tool for text manipulation. It’s been shown how methods like re.sub() and re.subn() can perform tasks such as removing digits, reformatting dates, and efficiently replacing substrings. It’s been demonstrated that compiling regular expressions, using precise special characters, employing non-capturing groups, and applying raw strings can significantly enhance efficiency. Additionally, the use of ‘re.IGNORECASE’ for case insensitivity adds to the flexibility of regex in Python.