In the realm of Python programming, managing lists effectively is a critical skill. One common task is removing an item from a list by its index. It’s a seemingly simple operation, but it holds a few surprises for beginners and seasoned coders alike.
This article dives into the nuances of the ‘remove item from list python by index’ operation, offering clear, concise explanations. It’s an essential read for anyone looking to sharpen their Python skills or those seeking a refresher on list manipulations.
Remove Item from List Python by Index
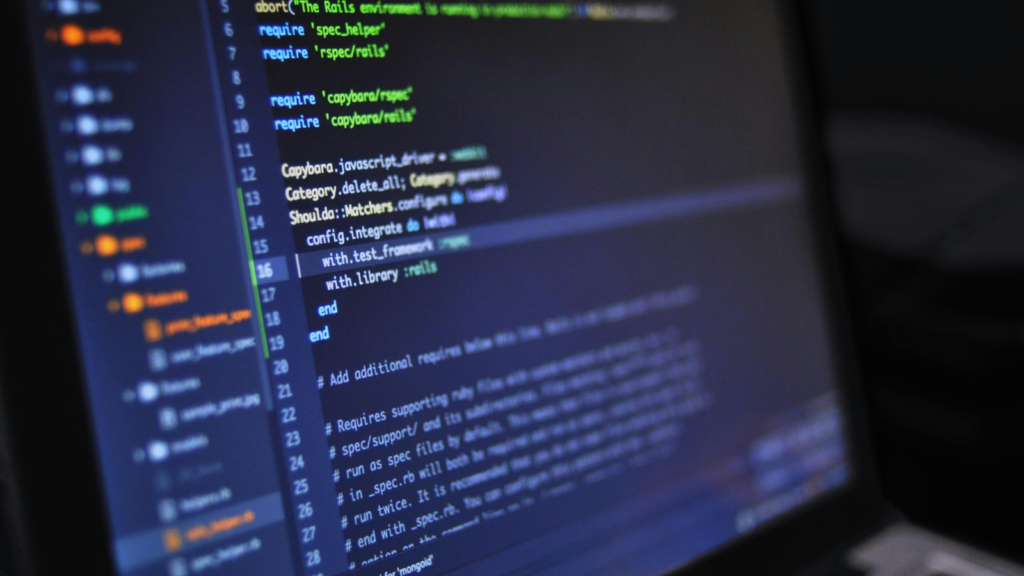
In Python, removing an item from a list by its index entails the utilization of a specific method, list.pop(index). This method enables efficient extraction and elimination of elements from lists using their respective indexes. For instance, if developers have a list called ‘fruits’, containing [‘apple’, ‘banana’, ‘cherry’], and they wish to remove ‘banana’, they’d invoke the .pop() method with the index number of ‘banana’ (which is 1) as the argument, like so: fruits.pop(1). Post execution, the ‘fruits’ list adjusts to [‘apple’, ‘cherry’].
Exercise caution, because Python lists use zero-based indexing. Therefore, the first item resides at position 0, second at 1, and so forth. Misinterpretation of this rule can lead to unforeseen manipulations – a pitfall easily avoided with an astute understanding of list operations in Python.
Methods to Remove Items by Index in Python
Python offers alternatives to the list.pop() method for users wishing to remove items from a list by index. Employing these can exhibit better alignment to various coding requirements.
- Del statement: It facilitates item deletion from a list by index. Following usage del list[index] will remove the item at the specified index.
Example:
# list before deletion
fruits = [‘apple’, ‘banana’, ‘cherry’, ‘date’, ‘elderberry’]
print(fruits)
deleting itemdel fruits[2]list after deletionprint(fruits)
In this example, the ‘cherry’ gets deleted as it’s at the 2nd index (zero-based).
- List comprehension: It’s beneficial when wanting to remove items at multiple indexes. A new list gets created excluding the items at the specified indexes.
Example:
# list before deletion
fruits = [‘apple’, ‘banana’, ‘cherry’, ‘date’, ‘elderberry’]
print(fruits)
deleting itemsfruits = [i for j, i in enumerate(fruits) if j not in (1,2)]list after deletionprint(fruits)
In this example, ‘banana’ and ‘cherry’ are deleted as they occupy the 1st and 2nd index, respectively.
Best Practices When Removing Items From a List
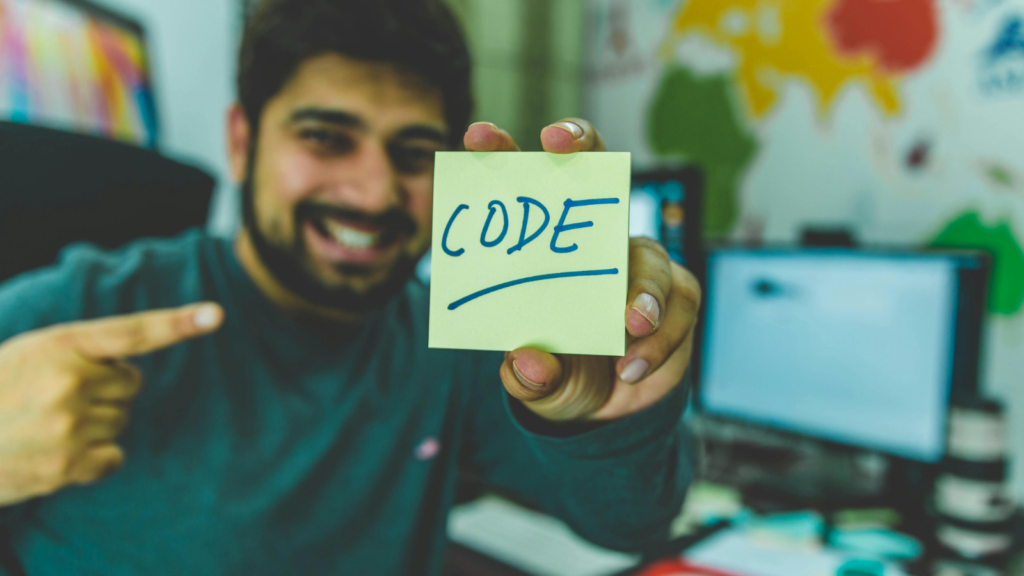
When looking to remove an item from list Python by index, always verify the list’s length. Determining the list’s length prevents inadvertent out-of-index errors. Python offers myriad methods to compute a list’s length, the most common being the len() function.
Unsurprisingly, programmers often overlook Python’s zero-based index. While working with list indices, always remember the first item’s position is 0, not 1. Ensuring this pivotal detail facilitates accurate removal of specific list items.
One widely regarded practice is the encapsulation of the list removal code within try-except blocks. This tactic allows the code to run even when certain exceptions occur, ensuring interrupted execution doesn’t dampen productivity.
Common Mistakes to Avoid
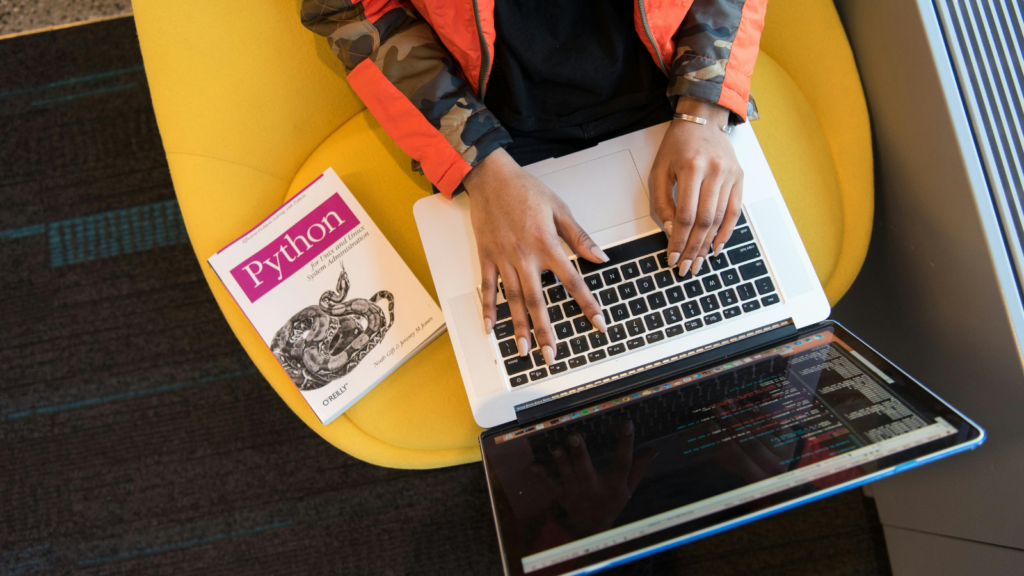
While managing lists in Python, specifically when you want to “remove item from list Python by index,” there exist critical errors to dodge. Mistaking index values – considering the first item as index 1 rather than index 0 – leads to unexpected results. Check the size of list before item removal; attempts to remove items beyond the actual length cause exceptions. Handling removal operations in try-except blocks provides error control. Additionally, avoid manipulating the list mid-loop. Concurrent deletion and iteration can cause skipped items or out-of-bounds errors, impacting the overall functionality. By steering clear of these common pitfalls, programmers ensure a competent, efficient approach to manipulating Python lists by index.
Mastering List Manipulation in Python
Mastering list manipulation in Python is crucial, and this post has shed light on how to remove items by index effectively. The use of list.pop() and the del statement, as well as list comprehension, have been demonstrated as valuable techniques. It’s also been underlined that using try-except blocks can be a smart move to prevent unexpected errors. Lastly, avoiding list manipulation during iteration has been stressed as a key to efficient and error-free Python programming.