In the vibrant world of Python programming, dictionaries are a vital tool. They’re incredibly versatile, but what happens when you need to modify them? Specifically, what if you need to perform a python dict remove key operation?
So, ready to dive into the depths of Python dictionaries and emerge with newfound knowledge? Let’s get started on this journey, and by the end, the concept of python dict remove key will be second nature to you.
Python Dict Remove Key
Python’s built-in function, dict.pop(key), serves in removing a key from a dictionary. This method executes a beneficial operation in Python dict remove key tasks. Utilization of this function requires a key as an argument. It returns the value of the removed key. An instance helps shed light on its usage:
python_dict = {‘apple’: 1, ‘orange’: 2, ‘banana’: 3}
removed_value= python_dict.pop(‘orange’)
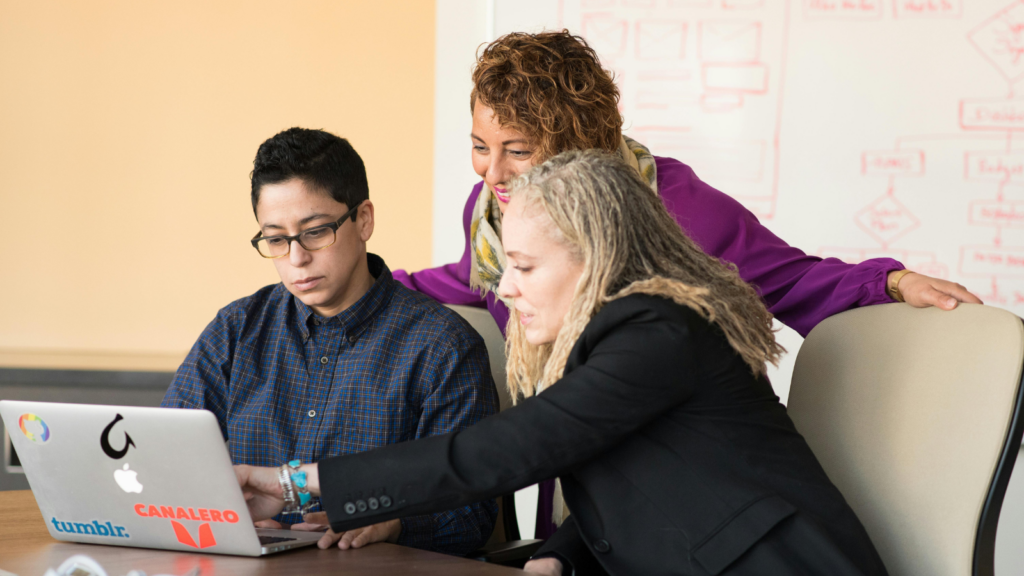
print(python_dict)
print(‘Removed value:’, removed_value)
Upon execution, it displays the modified dictionary, {‘apple’: 1, ‘banana’: 3}, alongside the removed value: `Removed value: 2.
Within python dict remove key procedures, if the specified key doesn’t exist in the dictionary, Python returns a KeyError. However, programmers can bypass this by providing a secondary argument — Python returns this argument instead if the key isn’t present. For instance:
value = python_dict.pop(‘mango’, ‘No fruit by this name’)
print(value)
By running this code, Python returns ‘No fruit by this name’ since ‘mango’ isn’t present in the dictionary.
Methods to Remove a Key from a Python Dictionary
Over time, various methods have emerged for executing the python dict remove key operation. In this segment, we explore three predominant methods: dict.pop(key), del dict[key], and dict comprehension.
The dict.pop(key) & del dict[key] Method
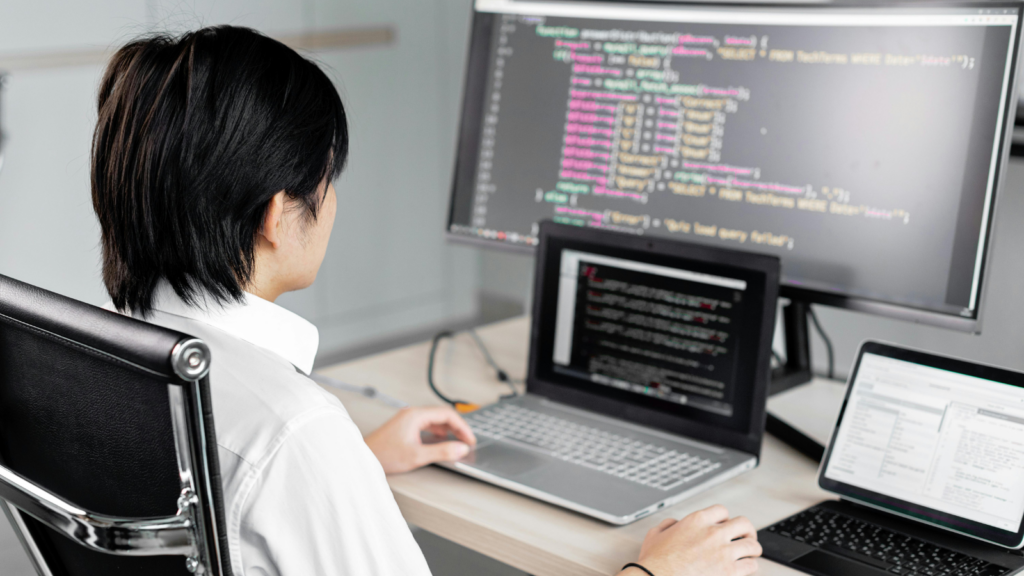
Existing in every Python dictionary, the dict.pop(key) method ensues a specified key’s removal while simultaneously returning the value. However, it triggers a KeyError, should the key not exist within the dictionary. Handling KeyError is essential to prevent application crashes, achievable by passing a secondary parameter to the dict.pop(key) method, known as a default value.
Offering a straightforward removal process, the del dict[key] method expunges a key from the Python dictionary directly. Unlike dict.pop(key), it doesn’t return the removed value, making it a suitable alternative when value retrieval remains unessential. It’s important to note the use of try and except to control for KeyError, as it’s just as liable to this exception as dict.pop(key).
The dict Comprehension Method
As Python’s most expressive approach, dict comprehension allows for key removal by constructing a new dictionary devoid of the key in question. Though it consumes more memory, its ability to remove multiple keys in a single line of code makes it a valuable tool for Python programmers.
Common Mistakes and How to Avoid Them
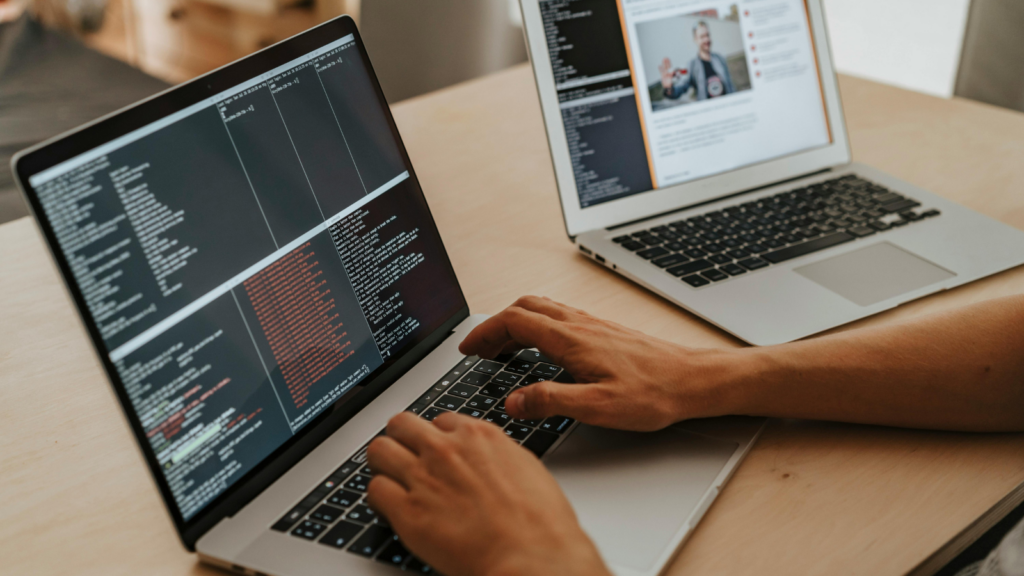
In Python, removing keys from dictionaries can involve typical missteps. One prevalent error intervenes when a developer tries to eliminate a key that doesn’t exist, causing a KeyError. It’s where understanding the dict.pop(key, default_value) function gains significance. This function, instead of just dict.pop(key), safeguards against KeyErrors. If the key isn’t present, it returns the provided default_value, preventing potential application crashes.
Another common mistake pertains to the improper use of dictionary comprehension. Developers tend to misuse this method when tackling complex scenarios. Instead of restructuring dictionaries and removing keys, they inadvertently add unnecessary loops or duplicate keys. It’s a good practice to maintain a clear comprehension structure and ensure to specify the condition accurately when coding with the python dict remove key concept.
Essential Skill for Any Programmer
Mastering Python’s dictionary key removal techniques is an essential skill for any Python programmer. It’s not just about knowing the methods, but also about using them efficiently and avoiding common pitfalls. Remember, dict.pop(key) and del dict[key] are your go-to methods, while dict comprehension offers more flexibility. But don’t forget the importance of handling KeyError occurrences. Using dict.pop(key, default_value) can be your safety net to prevent crashes.